When growing decentralized functions (dapps) and different Web3 platforms, you’ll rapidly discover that you simply want environment friendly methods of interacting and speaking with numerous blockchain networks. A method of doing so is establishing blockchain listeners for monitoring related good contract occasions. Nevertheless, the million-dollar query is, how do you create blockchain listeners? A distinguished choice is ethers.js, which is an Ethereum JavaScript library. If you wish to learn to use ethers.js to take heed to good contract occasions, be part of us on this tutorial as we present you precisely how to take action!
Earlier than we present you how you can use ethers.js to take heed to occasions, let’s return to fundamentals and discover the weather of this library in additional element. From there, we’ll present a fast introduction to occasions on Ethereum – which we’re utilizing synonymously with “ethers.js occasions” – supplying you with a greater thought of what we wish to monitor. After getting familiarized your self with ethers.js and Ethereum occasions, we are going to present you how you can arrange a blockchain listener with ethers.js to watch on-chain occasions. Lastly, we are going to prime all the things off with a comparability between ethers.js and Moralis’ Web3 Streams API!
If you happen to already know how you can arrange blockchain listeners with ethers.js, you must take a look at extra Moralis content material right here on the Web3 weblog. As an example, take a look at the last word Web3 py tutorial or be taught all you want concerning the Sepolia testnet! What’s extra, earlier than transferring additional on this article, enroll with Moralis instantly! Creating an account is free, and as a member, you’ll be able to really leverage the facility of blockchain expertise!
What’s Ethers.js?
Ethers.js is an Ethereum JavaScript (JS) library launched in 2016 by Richard Moore. It’s one in every of at this time’s most well-used Web3 libraries, that includes tens of millions of downloads. Like conventional libraries, ethers.js additionally consists of a set of prewritten snippets of code used to carry out widespread programming duties. Nevertheless, a big distinction between standard libraries and ethers.js is that the latter is blockchain-based. Accordingly, this library makes it simpler for builders to work together with the Ethereum community.
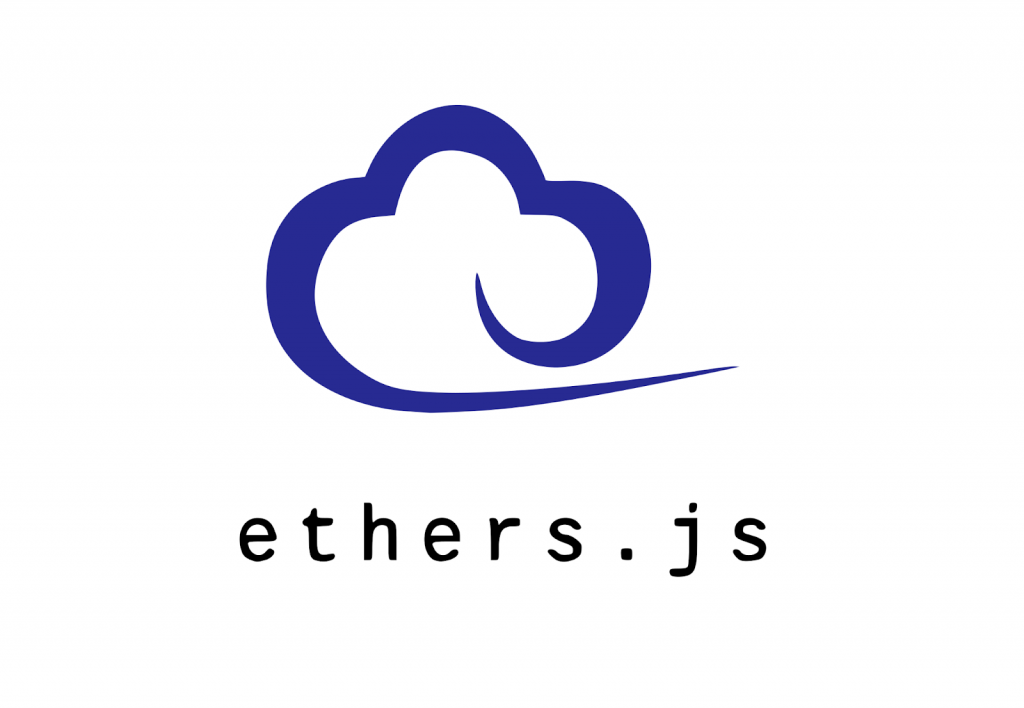
The library was initially designed to work with ”ethers.io”; nevertheless, since its launch, it has turn into a extra general-purpose library. Furthermore, ethers.js contains a user-friendly API construction and is written in TypeScript. In consequence, it is among the prime decisions amongst rising Web3 builders, as ethers.js is easy and intuitive to make use of.
However, to provide you a extra profound understanding of the library’s utility, allow us to briefly record among the foremost options of ethers.js:
ENS – Ethers.js helps Ethereum Title Service (ENS), that means ENS names are dealt with as first-class residents. Take a look at Instances – The library options an intensive assortment of take a look at circumstances, that are actively up to date and maintained. Dimension – Ethers.js is small, solely 284 KB uncompressed and 88 KB compressed.
Moreover, ethers.js has 4 core modules: “ethers.utils“, “ethers.wallets“, “ethers.supplier“, and “ethers.contract“. These 4 elements are important for the library’s utility programming interface (API). Nevertheless, if you wish to be taught extra about these, please take a look at the next information answering the query, ”what’s ethers.js?” in additional element. You may additionally wish to take into account trying out extra Web3 libraries. In that case, learn our article on Web3.js vs ethers.js!
Occasions on Ethereum – What are Ethers.js Occasions?
Good contracts on the Ethereum blockchain networks typically emit numerous occasions every time one thing occurs inside them based mostly on the code. What’s extra, these occasions are a sort of sign emitted from contracts to inform dapps and builders that one thing of relevance has transpired. Accordingly, builders and platforms can use these indicators to speak.
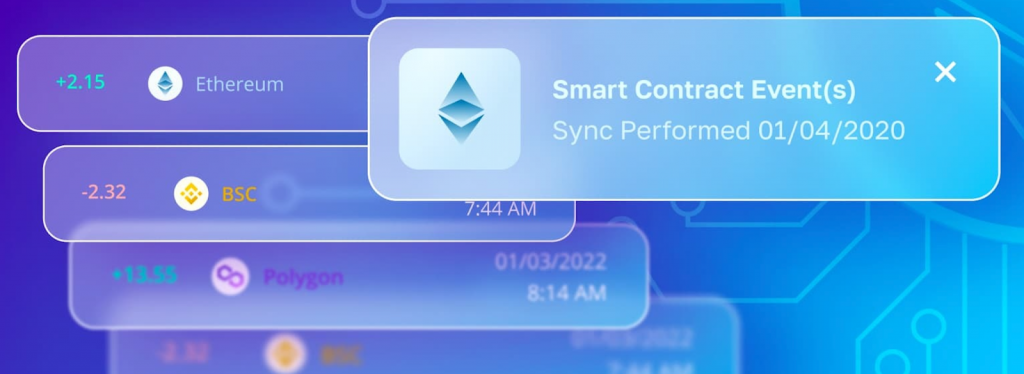
To make this definition extra easy, allow us to take a more in-depth have a look at the ERC-20 token normal for instance. All tokens implementing this good contract normal autonomously emit a ”switch” occasion every time they’re traded, and it’s potential to arrange blockchain listeners monitoring these occasions. What’s extra, this is just one instance, and most good contracts emit occasions based mostly on different occurrences alike. Furthermore, these are the occasions that we’re moreover calling “ethers.js occasions”.
So, as you might need figured your self, it is very important be capable of seamlessly pay attention to those ethers.js occasions in actual time. Additional, a technique of doing so is thru blockchain listeners. Nevertheless, how do you create one? If you’re searching for the reply to this query, be part of us within the subsequent part, the place we are going to present you how you can use ethers.js to take heed to blockchain occasions!
How Can Builders Use Ethers.js Occasions?
Now that what ethers.js occasions are, the massive query is, how do you utilize them? To adequately reply this query, the next part supplies an ethers.js instance the place we are going to present you how you can create a blockchain listener for monitoring the USD coin (USDC) good contract. Particularly, you’ll learn to monitor the good contract’s switch occasions.
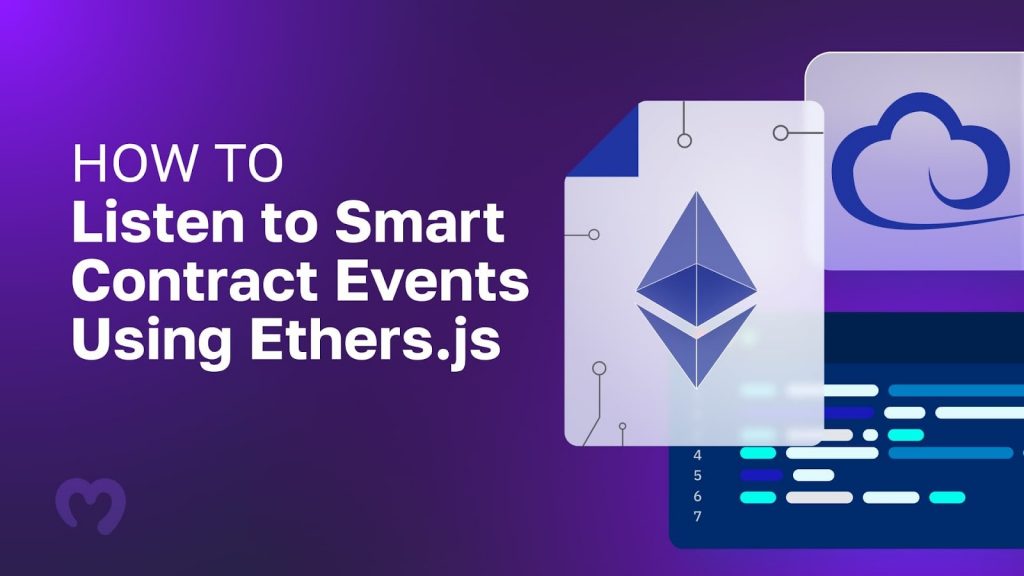
That stated – earlier than masking the code for the blockchain listener – you will need to first arrange a NodeJS venture. From there, create two new information: ”.env” and ”abi.json”. Furthermore, it is advisable to make the most of a node supplier when utilizing ethers.js to take heed to blockchain occasions. So, on this occasion, we shall be utilizing Alchemy. Consequently, you will need to add your Alchemy key as an setting variable to the ”.env” file.
Subsequent, together with the supplier key, it is advisable to add the USDC contract utility binary interface (ABI) to the ”abi.json” file. If you happen to need assistance discovering the ABI of any Ethereum-based contracts, take a look at Etherscan.
Lastly, to conclude the preliminary setup means of the NodeJS venture, it is advisable to set up a couple of dependencies. As such, open a brand new terminal and run the next command:
npm i ethers dotenv
Easy methods to Set Up an Ethers.js Blockchain Listener
Now that you’ve arrange the NodeJS venture, this part focuses on the ethers.js blockchain listener. As such, to proceed, create a brand new JavaScript file referred to as ”index.js”. Open the file and begin by including the next three strains of code on the prime:
const ethers = require(“ethers”);
const ABI = require(“./abi.json”);
require(“dotenv”).config();
The three strains from the code snippet above point out that ”index.js” makes use of the ethers.js library, together with importing the contract ABI and the setting variables from ”.env”. From there, the subsequent step is including an asynchronous operate we’re going to name ”getTransfers()”:
async operate getTransfer(){
const usdcAddress = “0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48”; ///USDC Contract
const supplier = new ethers.suppliers.WebSocketProvider(
`wss://eth-mainnet.g.alchemy.com/v2/${course of.env.ALCHEMY_KEY}`
);
const contract = new ethers.Contract(usdcAddress, ABI, supplier);
contract.on(“Switch”, (from, to, worth, occasion)=>{
let transferEvent ={
from: from,
to: to,
worth: worth,
eventData: occasion,
}
console.log(JSON.stringify(transferEvent, null, 4))
})
}
This operate accommodates the logic for the ethers.js blockchain listener. Consequently, we are going to break down the code from prime to backside. Initially, on the primary two strains of the ”getTransfers()” operate, the code creates two variables: ”usdcAddress” and ”supplier”. For the primary one, you wish to specify the contract tackle. For the latter, you will need to decide the node supplier utilizing your setting variable key.
Subsequent, the code creates a brand new ”contract” object by using the ”usdcAddress”, ”ABI”, and ”supplier” variables by passing them as arguments for the ”ethers.Contract()” operate. From there, the code units the listener utilizing the ”contract” object, specifying ”Switch” occasions as the subject of curiosity. Lastly, the code console logs the outcomes!
However, the whole code of the ”index.js” file ought to now look one thing like this:
const ethers = require(“ethers”);
const ABI = require(“./abi.json”);
require(“dotenv”).config();
async operate getTransfer(){
const usdcAddress = “0xA0b86991c6218b36c1d19D4a2e9Eb0cE3606eB48”; ///USDC Contract
const supplier = new ethers.suppliers.WebSocketProvider(
`wss://eth-mainnet.g.alchemy.com/v2/${course of.env.ALCHEMY_KEY}`
);
const contract = new ethers.Contract(usdcAddress, ABI, supplier);
contract.on(“Switch”, (from, to, worth, occasion)=>{
let transferEvent ={
from: from,
to: to,
worth: worth,
eventData: occasion,
}
console.log(JSON.stringify(transferEvent, null, 4))
})
}
getTransfer()
Easy methods to Run the Script
With all of the code added to the ”index.js” file, all that continues to be is working the script. To take action, open a brand new terminal and run the next command:
node index.js
When you execute the command above, the code will return USDC transactions information in your console, and it’ll look one thing like this:
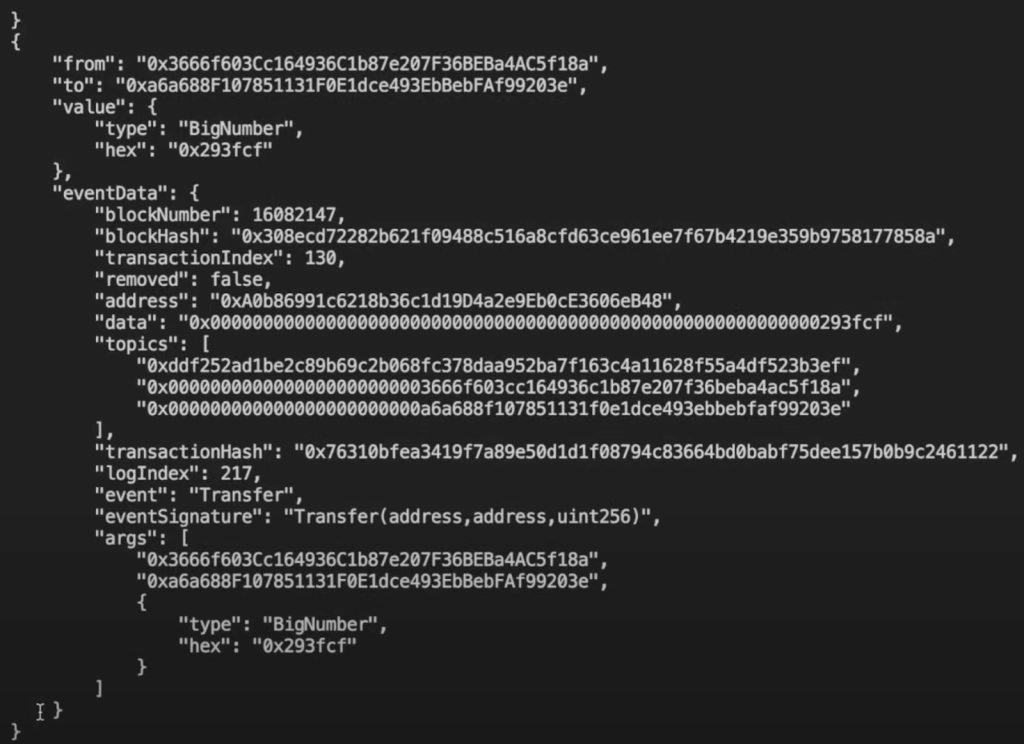
Working the script returns an abundance of knowledge, together with the to and from addresses, occasion information, and rather more. Sadly, the response above doesn’t comprise parsed information, and we can not, as an example, decide the origin of the knowledge. In conclusion, utilizing ethers.js for listening to blockchain occasions is a superb begin, however it leaves extra to be desired. So, are there any ethers.js alternate options?
Is There an Various to Ethers.js Occasions?
Despite the fact that ethers.js is an efficient choice for establishing blockchain listeners for monitoring good contract occasions, it’s not excellent. Consequently, it’s price contemplating different alternate options as effectively. As an example, probably the most distinguished examples is Moralis’ Streams API!
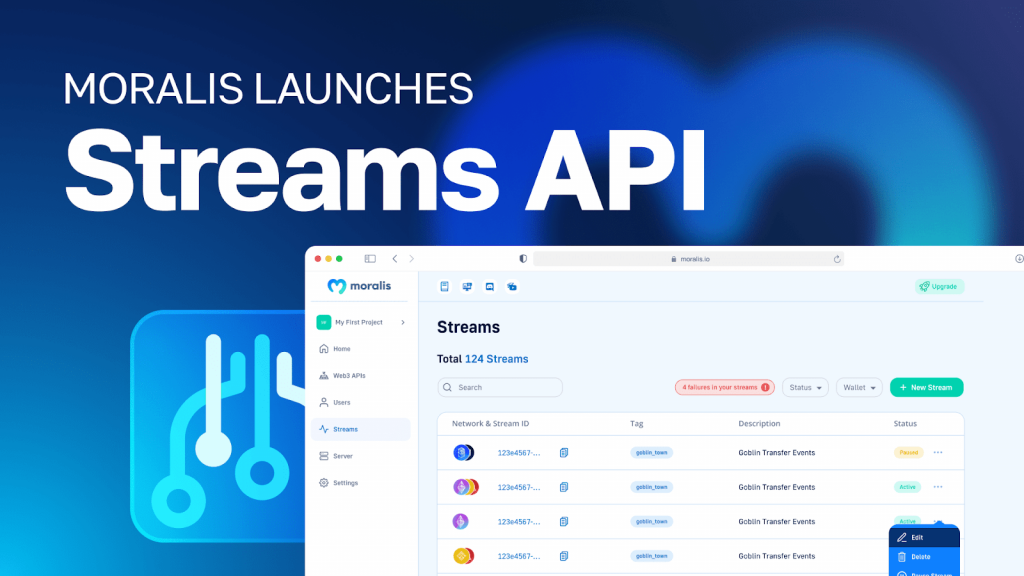
With the Streams API, you’ll be able to effortlessly stream on-chain information into the backend of your blockchain tasks through Web3 webhooks. By means of this Moralis function, you’ll be able to arrange streams to obtain Moralis webhooks every time an tackle receives, swaps, or stakes an asset, a battle begins in your blockchain sport, or some other good contract occasions set off based mostly in your filters!
What’s extra, by way of the accessibility of Moralis, you’ll be able to arrange your personal streams in 5 easy steps:
Present a wise contract addressApply filters specifying when to obtain webhooksSelect the chain(s) you wish to monitorAdd your webhook URLReceive webhooks
However, allow us to discover how Moralis’ Web3 Streams API is healthier than ethers.js!
How the Web3 Streams API is Higher Than Ethers.js
Ethers.js is an efficient different for setting blockchain listeners to watch good contract occasions. Nevertheless, when you begin working with this library, you’ll rapidly discover its limitations. As such, it’s price contemplating different choices like Moralis’ Streams API as a substitute. To provide you a greater thought of why that is, we have now summarized the similarities and variations between ethers.js and Moralis down under:

With this fast overview, you’ll be able to immediately see that Moralis supplies all the things ether.js does and extra when establishing blockchain listeners. Nevertheless, simply trying on the factors made within the desk above might be considerably cryptic. Subsequently, allow us to study and break down the desk for you within the following sub-section to supply an in-depth evaluation of the variations between ethers.js and the choice the place customers can arrange Web3 streams utilizing the Streams API!
Ethers.js vs Web3 Streams
The desk above reveals that each ethers.js and Moralis streams let you monitor real-time occasions. Each choices help a number of chains, that means you’ll be able to monitor occasions for various blockchain networks. Nevertheless, whereas that covers the similarities, how do these two choices differ?

First, Moralis provides 100% reliability. However what does this imply? As you may bear in mind from the ”How Can Builders Use Ethers.js Occasions?” part, it is advisable to present a separate node supplier when utilizing ethers.js. This may be problematic, as it’s troublesome to know if the nodes maintained by the supplier will keep operational indefinitely. Nevertheless, this isn’t the case with Moralis, as you have got a single tech stack and at all times obtain real-time alerts by way of webhooks as a substitute.
You possibly can moreover filter occasions with Moralis’ Web3 Streams API. Consequently, you’ll be able to goal occasions and solely obtain webhooks for the on-chain information you require. You can too pool contracts right into a single stream, which is inconceivable when utilizing ethers.js. With ethers.js, you’ll as a substitute have to arrange separate blockchain listeners for brand new contracts.
Lastly, you’ll be able to take heed to pockets addresses when utilizing Moralis. This implies you’ll be able to obtain webhooks every time a pockets performs a specific motion. Furthermore, together with the aforementioned advantages, the info acquired from Moralis webhooks is parsed. As such, you don’t want to fret about extra formatting and processing. This additional signifies that the info is able to use ”straight out of the field” in your Web3 tasks.
However, take a look at our article on ethers.js vs Web3 streams for extra details about the variations. You may additionally wish to watch the video down under, exhibiting you how you can arrange blockchain listeners utilizing each ethers.js and Moralis’ Web3 Streams API:
Ethers.js Occasions – Abstract
When you have adopted alongside this far, you now know how you can take heed to good contract occasions with ethers.js. On this tutorial, we taught you how you can create a blockchain listener for monitoring the switch occasion of the USDC good contract. In doing so, the article confirmed you how you can arrange a NodeJS venture, add the code for the blockchain listener, and run the script. Now you can use the identical elementary ideas to watch any good contract occasions utilizing ethers.js in your future blockchain tasks!
If you happen to discovered this ethers.js instance tutorial useful, take into account trying out extra Moralis content material. As an example, learn our article on how you can get all transfers of an NFT. In that information, you get to discover the accessibility of Moralis’ NFT API, which is among the many Web3 APIs provided by Moralis. Different distinguished examples are the Token API, Solana API, EVM API, and so on. Thanks to those instruments, Moralis presents the quickest method to construct a Web3 app!
Consequently, it doesn’t matter what Web3 improvement endeavors you embark on, enroll with Moralis, as that is how one can absolutely leverage the facility of blockchain expertise!