[ad_1]
How do you program good contracts and implement them? Particularly, how do you accomplish that for the Solana community? Observe alongside on this article as we display a three-step means of the way to full the above duties. The strains of code that may make up your Solana good contract, or Solana program, will seem like this:
use solana_program::{
account_info::AccountInfo,
entrypoint,
entrypoint::ProgramResult,
pubkey::Pubkey,
msg,
};
entrypoint!(process_instruction);
pub fn process_instruction(
program_id: &Pubkey,
accounts: &[AccountInfo],
instruction_data: &[u8]
) -> ProgramResult {
msg!(“Howdy, world!”);
Okay(())
}
However the strains of code above are ineffective until you will have the required conditions set in place. Thus, learn on as we break down the method step-by-step on establishing the conditions, establishing the contract, and likewise offering you with a video walkthrough to finalize the method. Because of this, you’ll perceive the way to program good contracts and implement them on Solana!
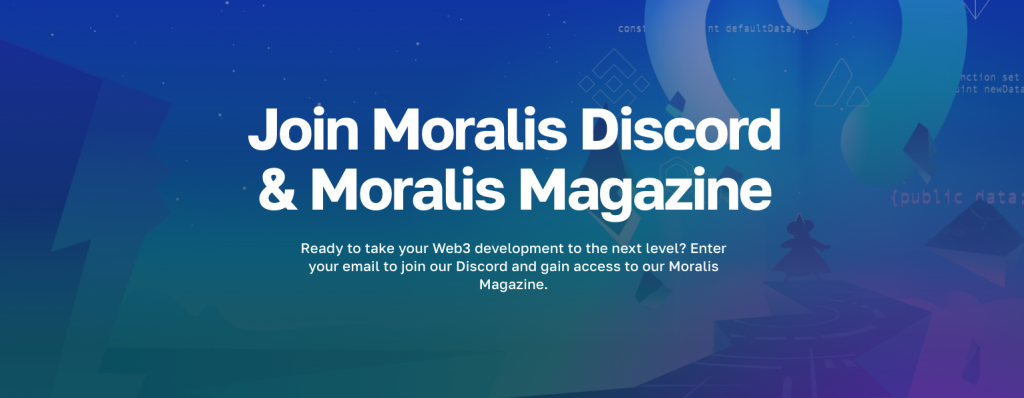
Overview
Transferring ahead, we’ll first be sure that you perceive what good contracts are. Then, we’ll concentrate on the programming languages that you will want to make use of to program good contracts. That is the place we’ll encounter the primary main distinction between Solana and EVM-compatible chains. In spite of everything, programming good contracts on Solana requires a special strategy than Ethereum programming. Furthermore, on this article, we’ll concentrate on the Solana community. But, we’ll take a look at some good contract examples for each Ethereum and Solana to make sure you correctly perceive their key elements. Final however not least, we’ll tackle a step-by-step tutorial exhibiting the way to program good contracts on Solana. That is additionally the place you’ll use the last word Solana API dropped at you by Moralis. Therefore, make sure that to have your free Moralis account prepared.
Introduction to Good Contracts
When you take a look at the picture above, you will note Ethereum’s emblem. That’s as a result of Ethereum was the primary programmable chain and, thus, the community that got here up with the idea of good contracts. Nonetheless, today, we have now a number of respected programmable blockchains, a lot of which assist good contracts. Furthermore, in terms of EVM-compatible blockchains, “good contracts” is the proper time period. However, in terms of good contracts on Solana, they’re technically referred to as “packages”.
So, whether or not we speak about “good contracts” or on-chain “packages”, they’re primarily one and the identical. Till we concentrate on the way to program good contracts on Solana or different chains, the variations don’t actually matter. In spite of everything, they’re each items of software program operating on a blockchain designed to set off particular pre-defined actions when corresponding circumstances are met. As such, good contracts have the facility to create trustless contracts and eradicate intermediaries. In flip, there are numerous use instances the place these on-chain packages are poised to make an enormous distinction sooner or later.
Moreover, it’s price stating that there’s a wise contract behind each on-chain transaction. Therefore, all token (fungible and non-fungible) minting is completed by deploying good contracts. Additionally, notice that there are particular requirements that good contracts should adjust to. As an illustration, on the Ethereum community and EVM-compatible chains, ERC-20, ERC-721, and ERC-1155 good contracts are used to mint tokens and management their transactions. As well as, these requirements additionally allow devs to make use of verified good contract templates.
Now that you recognize what good contracts or on-chain packages are, we will concentrate on the programming languages used to create them. That’s the place the good contract programming process differs between Solana and EVM-compatible chains.
What Programming Language is Used for Good Contracts?
When you resolve to jot down good contracts for Ethereum or different EVM-compatible chains, you’ll use the Solidity or Vyper programming languages. Among the many two choices, Solidity is much extra widespread. As such, there are extra assets, extra good contract templates, and so on., for Solidity. Thus, for those who resolve to develop initiatives for EVM-compatible chains, we encourage you to start out with Solidity.
However, if you wish to learn to program good contracts on Solana from scratch, you’ll be able to’t use Solidity. The programming languages for the Solana chain are Rust, C, and C++, with Rust being the popular language amongst builders. Therefore, that is the language we’ll use within the “the way to program good contracts on Solana” tutorial beneath.
It’s additionally price noting that every program on Solana has a singular and identifiable ID to a singular proprietor that may improve the executable code:
Nonetheless, except for completely different programming languages, good contract improvement on Solana additionally requires completely different instruments. As an illustration, you’ll be able to’t use the MetaMask Web3 pockets to pay transaction charges on Solana. Additionally, you’ll be able to’t use Hardhat or Remix to compile, deploy and confirm good contracts. As a substitute, you could use instruments designed for Solana programming. For one, you want the Solana CLI, and also you additionally want to make use of a Solana Web3 pockets. You’ll study extra in regards to the instruments wanted to create Solana packages within the tutorial beneath.
Be aware: If you wish to study Rust programming, Moralis Academy has a fantastic course.
Good Contract Examples
Relating to discovering good contract examples, Ethereum presents far more templates. In spite of everything, the Ethereum blockchain has been round for a few years and has a a lot bigger neighborhood. Nonetheless, this additionally implies that Solana presents numerous alternatives for brand spanking new contracts to be developed.
Furthermore, good contracts can be utilized for numerous functions. Nonetheless, nearly all of good contract examples concentrate on these use instances:
Crypto token creation (minting) and transactions Doc preservation and accessibilityAdministrative funds and billingPayrollsTaxationPensionsInsuranceBill paymentsStatistics collationHealth and agricultural provide chainsReal property and crowdfundingIdentity administration
That stated, we’ll check out the strains of code for a Solana instance program within the subsequent part. Beneath, we concentrate on two token good contracts for Ethereum. Additionally, notice that you could find numerous verified EVM-compatible good contracts at OpenZeppelin.
Here’s a primary ERC-20 good contract:
// contracts/GLDToken.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC20/ERC20.sol”;
contract GLDToken is ERC20 {
constructor(uint256 initialSupply) ERC20(“Gold”, “GLD”) {
_mint(msg.sender, initialSupply);
}
}
Right here’s an instance of a primary ERC-721 good contract:
// contracts/GameItem.sol
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “@openzeppelin/contracts/token/ERC721/extensions/ERC721URIStorage.sol”;
import “@openzeppelin/contracts/utils/Counters.sol”;
contract GameItem is ERC721URIStorage {
utilizing Counters for Counters.Counter;
Counters.Counter personal _tokenIds;
constructor() ERC721(“GameItem”, “ITM”) {}
operate awardItem(deal with participant, string reminiscence tokenURI)
public
returns (uint256)
{
uint256 newItemId = _tokenIds.present();
_mint(participant, newItemId);
_setTokenURI(newItemId, tokenURI);
_tokenIds.increment();
return newItemId;
}
}
Tutorial: Methods to Program Good Contracts on Solana
Observe the steps lined herein and use the video beneath for extra particulars on the way to full this tutorial. In brief, studying the way to program good contracts on Solana entails the next three steps:
Set up Rust and the Solana CLICreate and Deploy an Instance Solana ProgramCreate a Testing Dapp to Name the Good Contract
Concerning step one, you could mainly observe the steps outlined within the Solana docs. Nonetheless, issues get a bit extra fascinating within the second step. After all, our instance Solana program will probably be fairly simple; nonetheless, when you’ve got massive concepts, that is the place you’ll be able to create all kinds of good contracts. Although, deploying your good contract is identical for the only and essentially the most advanced strains of code.
So far as the third step goes, you’ll have an opportunity to create an precise dapp. Utilizing our repo, you are able to do this in minutes. Nonetheless, it’s also possible to use the last word Ethereum boilerplate as a place to begin and apply the tweaks to create your distinctive dapp. Furthermore, we’ll present you the way to acquire your Moralis Web3 API key. In spite of everything, the latter can also be the gateway to the last word Solana API. Lastly, you’ll be capable of make calls to your instance program out of your dapp.
Step 1: Set up Rust and the Solana CLI
Be aware: When you choose to observe together with video directions, make sure that to make use of the shifting image beneath, beginning at 2:09. Furthermore, in case this isn’t your first rodeo with Solana, and you have already got these two instruments put in, be happy to skip this step.
Begin by opening a brand new Unix terminal and enter this command:
curl –proto ‘=https’ –tlsv1.2 -sSf https://sh.rustup.rs | sh
After operating the above command, amongst a number of set up varieties, you’ll want to pick one sort. For the sake of this tutorial, we encourage you to go along with the default choice. As such, merely enter “1”:
The method might take a while to complete, however that’s it for the Rust set up. Therefore, you’ll be able to proceed with the Solana CLI set up (3:26). First, enter this command:
sh -c “$(curl -sSfL https://launch.solana.com/secure/set up)”
With the set up completed, set the “env path“. You are able to do the latter in your terminal logs:
Transferring on, you could create a brand new native Solana key pair (native pockets). As such, enter these two instructions:
mkdir ~/my-solana-walletsolana-keygen new –outfile ~/my-solana-wallet/my-keypair.json
Then, you’ll be capable of purchase your pockets deal with with the next:
solana deal with
Subsequent, you additionally must set the devnet cluster:
solana config set –url https://api.devnet.solana.com
Final however not least, you could get some check SOL to cowl the transactions on Solana devnet. That is the command that may airdrop you one SOL:
solana airdrop 1
The above command line additionally concludes the preliminary setup.
Step 2: Create and Deploy an Instance Solana Program
Be aware: Alternatively, use the video beneath, beginning at 5:07.
This step is the gist of the “the way to program good contracts on Solana” quest. We’ll be utilizing the Visible Studio Code (VSC) IDE, and we encourage you to do the identical to keep away from any pointless confusion. After all, every other IDE may also do the trick.
Inside VSC, create a brand new folder (our instance: “Solana-contract”) and launch a brand new terminal:
Then, create a brand new Rust venture utilizing the next command:
cargo init hello_world –lib
The above command will add a Cargo library to your venture’s folder:
Subsequent, go into the “hello_world” folder. You are able to do this by coming into the next command:
cd hello_world
As soon as within the above folder, proceed by updating the strains of code within the “Cargo.toml” file. Merely copy-paste the next strains of code:
[lib]
title = “hello_world”
crate-type = [“cdylib”, “lib”]
That is what it seems like in VSC:
Then, open the “lib.rs” file contained in the “src” folder and delete its content material. Transferring ahead, add the Solana program bundle by coming into the command beneath:
cargo add solana_program
Traces of Code for an Instance Solana Program
At this level, you will have all the pieces prepared so as to add the strains of code that may make up your Solana good contract (Solana program). As such, paste the next strains of code into “lib.rs:
use solana_program::{
account_info::AccountInfo,
entrypoint,
entrypoint::ProgramResult,
pubkey::Pubkey,
msg,
};
entrypoint!(process_instruction);
pub fn process_instruction(
program_id: &Pubkey,
accounts: &[AccountInfo],
instruction_data: &[u8]
) -> ProgramResult {
msg!(“Howdy, world!”);
Okay(())
}
Be aware: For the code walkthrough, use the video beneath.
With the above code in place, you’ll be able to proceed by constructing the venture. As such, enter this command into your terminal:
cargo build-bpf
As soon as the above command builds the venture, you’ll be able to deploy your Solana program utilizing the “solana program deploy” command. You have to complement this command with a goal path. Accordingly, enter this command:
solana program deploy ./goal/deploy/hello_world.so
Because of this, it’s best to get your program ID (you’ll use that in step three):
With the primary two steps underneath your belt, you already know the way to program good contracts on Solana. Therefore, it’s time to additionally learn to make the most of Solana packages.
Step 3: Create a Testing Dapp to Name the Good Contract
As promised, that is the place we hand you over to the video tutorial beneath, beginning at 9:38. Primarily, you simply must clone the code from GitHub, add your Moralis Web3 API key and your Solana program ID:
Clone our code:
Get hold of your Web3 API key (you want a Moralis account for that) and paste it into the “.env.native” file:
Paste your Solana program ID (finish of step two) into the designated line of the “HelloWorld.tsx” file:
Additionally, make sure that to mess around together with your new Solana dapp to check if it runs your good contract.
Lastly, right here’s the video tutorial that we’ve been referencing all through the article:
Methods to Program Good Contracts and Implement Them on Solana – Abstract
In as we speak’s article, you had an opportunity to learn to program good contracts on Solana. Nonetheless, earlier than we took on the tutorial, we lined the fundamentals. As such, you discovered that good contracts are executable clusters of code saved on a blockchain. These strains of code can learn and modify on-chain accounts. You additionally discovered that good contracts on Solana are known as packages. Moreover, we defined that Solana and EVM-compatible chains use completely different good contract programming languages. The latter use Solidity and Vyper, whereas Rust is the most well-liked choice for Solana. We additionally checked out some good contract examples. Final however not least, you had an opportunity to observe our lead and create and deploy your personal Solana program. Furthermore, you additionally had a possibility to expertise the facility of Moralis by creating your dapp in minutes.
If you’re excited by studying extra about Solana or Ethereum improvement, make sure that to go to the Moralis documentation, the Moralis YouTube channel, and the Moralis weblog. These assets include an ideal mixture of tutorials and easy explanations that may make it easier to turn into a Web3 developer free of charge. A few of our newest articles present you the way to add dynamic Web3 authentication to a web site, the way to use Firebase as a proxy API for Web3, the way to combine blockchain-based authentication, the way to create dapps utilizing a NodeJS Web3 instance, and way more.
Moreover, you could be excited by a extra skilled strategy to your crypto training. If that’s the case, Moralis Academy is what you want. There, you’ll turn into blockchain licensed, which can make it easier to go full-time crypto markedly faster.
[ad_2]
Source link