[ad_1]
On this article, we are going to exhibit construct a Polygon portfolio tracker by following the steps within the video above. Though the video focuses on Ethereum, we’ll goal the Polygon community just by updating the chain ID worth. Additionally, with two highly effective endpoints from Moralis, we are going to fetch the mandatory on-chain knowledge for our portfolio tracker. Let’s take a look at how easy it’s to make use of the 2 Web3 Knowledge API endpoints overlaying the blockchain-related side of a Polygon portfolio tracker:
const response = await Moralis.EvmApi.token.getWalletTokenBalances({
tackle,
chain,
});const tokenPriceResponse = await Moralis.EvmApi.token.getTokenPrice({
tackle,
chain,
});
To run the above two strategies, you additionally must initialize Moralis utilizing your Web3 API key:
Moralis.begin({
apiKey: MORALIS_API_KEY,
}
That’s it! It doesn’t need to be extra sophisticated than that when working with Moralis! Now, the above strains of code are the gist of the blockchain-related backend functionalities of portfolio trackers throughout a number of chains. Due to the cross-chain interoperability of Moralis, whether or not you want to construct a Polygon portfolio tracker or goal some other EVM-compatible chain, the above-presented code snippets get the job accomplished!

In fact, you have to implement the above strains of code correctly and add appropriate frontend parts. So, when you want to learn to do exactly that and create a clear Polygon portfolio tracker, be certain to observe our lead. However first, join with Moralis! In any case, your Moralis account is the gateway to utilizing the quickest Web3 APIs.
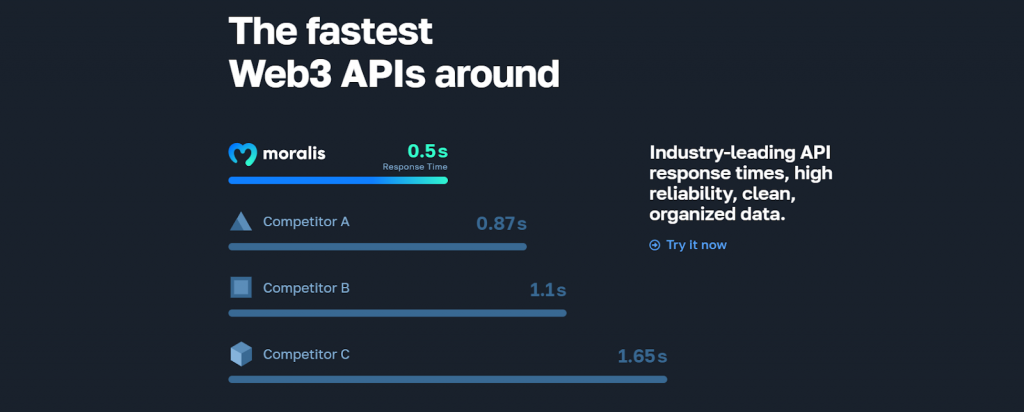
Overview
Since Polygon continues to be probably the most widespread EVM-compatible chains, we wish to exhibit construct a Polygon portfolio tracker with minimal effort. The core of at present’s article is our Polygon portfolio tracker tutorial. That is the place you’ll have an opportunity to clone our completed code for the Ethereum tracker and apply some minor tweaks to focus on the Polygon community. On this tutorial, you’ll be utilizing NodeJS to cowl the backend and NextJS for the frontend. So far as the fetching of on-chain knowledge goes, Moralis will do the trick with the above-presented snippets of code. You simply must retailer your Moralis Web3 API key in a “.env” file.
Apart from guiding you thru these minor tweaks, we can even stroll you thru crucial elements of the code. When you full the tutorial, you’ll even have an opportunity to discover different Moralis instruments you should use to create all kinds of highly effective dapps (decentralized functions). For instance, Moralis gives the Moralis Streams API, enabling you to stream real-time blockchain occasions straight into the backend of your decentralized software by way of Web3 webhooks!
All in all, after finishing at present’s article, you’ll be prepared to affix the Web3 revolution utilizing your legacy programming abilities!
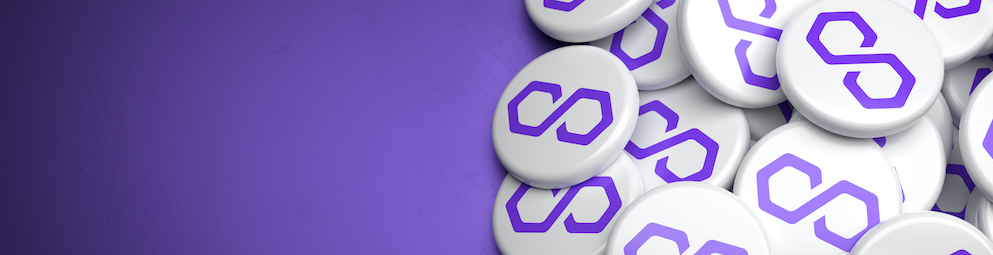
Now, earlier than you bounce into the tutorial, bear in mind to enroll with Moralis. With a free Moralis account, you achieve free entry to a few of the market’s main Web3 improvement assets. In flip, you’ll be capable to develop Web3 apps (decentralized functions) and different Web3 tasks smarter and extra effectively!
Tutorial: Find out how to Construct a Polygon Portfolio Tracker
We determined to make use of MetaMask as inspiration. Our Polygon portfolio tracker can even show every coin’s portfolio share, worth, and steadiness in a linked pockets. Right here’s how our instance pockets seems:
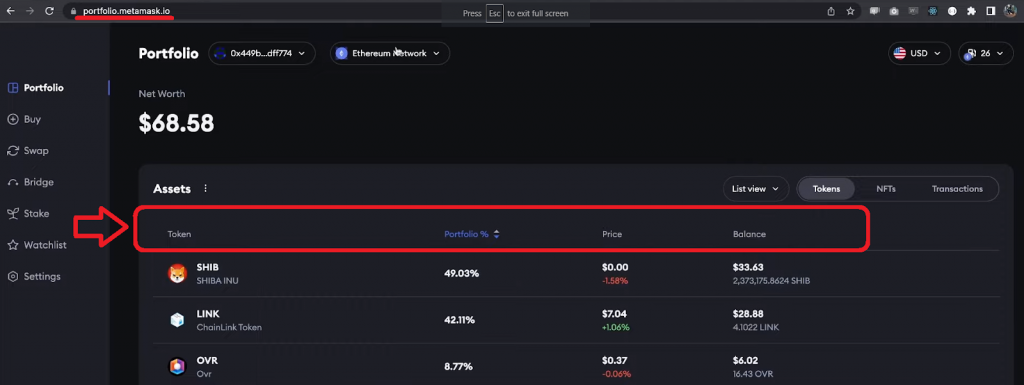
For the sake of simplicity, we’ll solely concentrate on the belongings desk on this article. With that mentioned, it’s time you clone our undertaking that awaits you on the “metamask-asset-table” GitHub repo web page:
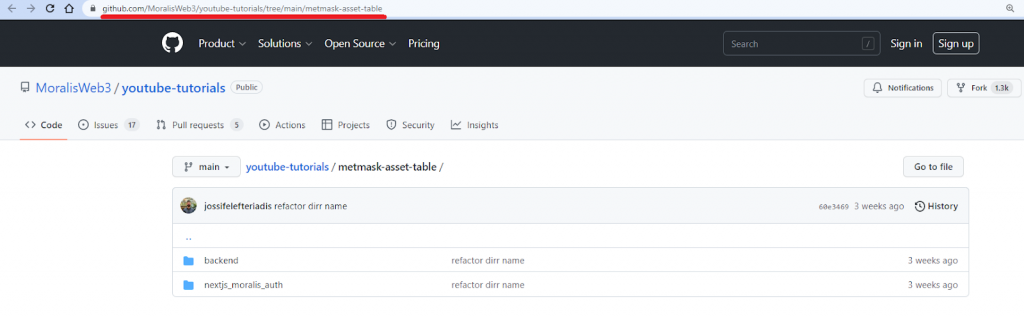
After cloning our repo into your “metamask-portfolio-table” undertaking listing, open that undertaking in Visible Studio Code (VSC). If we first concentrate on the “backend” folder, you may see it comprises the “index.js”, “package-lock.json”, and “package deal.json” scripts. These scripts will energy your NodeJS backend dapp.
Nonetheless, earlier than you may run your backend, you have to set up all of the required dependencies with the npm set up command. As well as, you additionally must create a “.env” file and populate it with the MORALIS_API_KEY environmental variable. As for the worth of this variable, you have to entry your Moralis admin space and replica your Web3 API key:
By this level, you need to’ve efficiently accomplished the undertaking setup. In flip, we will stroll you thru the principle backend script. That is additionally the place you’ll learn to change the chain ID to match Polygon.
Best Technique to Construct a Portfolio Tracker with Assist for Polygon
Should you concentrate on the backend “index.js” script, you’ll see that it first imports/requires all dependencies and defines native port 5001. The latter is the place you’ll be capable to run your backend for the sake of this tutorial. So, these are the strains of code that cowl these elements:
const specific = require(“specific”);
const app = specific();
const port = 5001;
const Moralis = require(“moralis”).default;
const cors = require(“cors”);
require(“dotenv”).config({ path: “.env” });
Subsequent, the script instructs the app to make use of CORS and Specific and to fetch your Web3 API key from the “.env” file:
app.use(cors());
app.use(specific.json());
const MORALIS_API_KEY = course of.env.MORALIS_API_KEY;
On the backside of the script, the Moralis.begin operate makes use of your API key to initialize Moralis:
Moralis.begin({
apiKey: MORALIS_API_KEY,
}).then(() => {
app.hear(port, () => {
console.log(`Listening for API Calls`);
});
});
Between the strains that outline the MORALIS_API_KEY variable and initialize Moralis, the script implements the 2 EVM API strategies offered within the intro. Listed below are the strains of code that cowl that correctly:
app.get(“/gettokens”, async (req, res) => {
attempt {
let modifiedResponse = [];
let totalWalletUsdValue = 0;
const { question } = req;
const response = await Moralis.EvmApi.token.getWalletTokenBalances({
tackle: question.tackle,
chain: “0x89”,
});
for (let i = 0; i < response.toJSON().size; i++) {
const tokenPriceResponse = await Moralis.EvmApi.token.getTokenPrice({
tackle: response.toJSON()[i].token_address,
chain: “0x89”,
});
modifiedResponse.push({
walletBalance: response.toJSON()[i],
calculatedBalance: (
response.toJSON()[i].steadiness /
10 ** response.toJSON()[i].decimals
).toFixed(2),
usdPrice: tokenPriceResponse.toJSON().usdPrice,
});
totalWalletUsdValue +=
(response.toJSON()[i].steadiness / 10 ** response.toJSON()[i].decimals) *
tokenPriceResponse.toJSON().usdPrice;
}
modifiedResponse.push(totalWalletUsdValue);
return res.standing(200).json(modifiedResponse);
} catch (e) {
console.log(`One thing went mistaken ${e}`);
return res.standing(400).json();
}
});
Trying on the strains of code above, you may see that we changed 0x1 with 0x89 for each chain parameters. The previous is the chain ID in HEX formation for Ethereum and the latter for Polygon. So, when you intention to construct a Polygon portfolio tracker, be certain to go together with the latter. You too can see that the getWalletTokenBalances endpoint queries the linked pockets. Then, the getTokenPrice endpoint loops over the outcomes supplied by the getWalletTokenBalances endpoint. That method, it covers all of the tokens within the linked pockets and fetches their USD costs. It additionally calculates the entire worth of the pockets by merely including the USD values of all tokens. Lastly, our backend script pushes the outcomes to the frontend shopper.
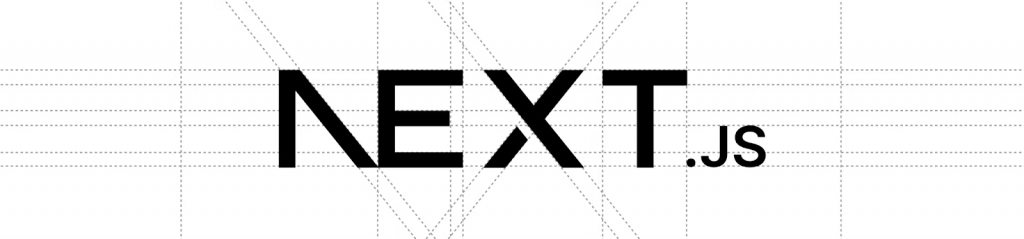
Frontend Code Walkthrough
As talked about above, you’ll create the frontend of your Polygon portfolio tracker dapp with NextJS. So, the “nextjs_moralis_auth” folder is actually a NextJS app. To make it work, you have to set up all dependencies utilizing the npm set up command. Nonetheless, ensure you cd into the “nextjs_moralis_auth” folder earlier than working the command. As soon as you put in the dependencies, you may run your frontend and mess around along with your new Polygon portfolio tracker. However since we wish you to get some further perception from this text, let’s take a look at essentially the most vital snippets of the code of the varied frontend scripts.
The “signin.jsx” script supplies the “Join MetaMask” button. As soon as customers join their wallets, the “consumer.jsx” script takes over. The latter gives a “Signal Out” button and renders the LoggedIn element, each contained in the Consumer operate:
operate Consumer({ consumer }) {
return (
<part className={kinds.primary}>
<part className={kinds.header}>
<part className={kinds.header_section}>
<h1>MetaMask Portfolio</h1>
<button
className={kinds.connect_btn}
onClick={() => signOut({ redirect: “/” })}
>
Signal out
</button>
</part>
<LoggedIn />
</part>
</part>
);
}
Should you take a look at the “loggedIn.js” element, you’ll see that it comprises two different parts: “tableHeader.js” and “tableContent.js“. As such, these two parts be certain the on-chain knowledge fetched by the above-covered backend is neatly offered on the frontend.
Portfolio Desk Elements
Right here’s a screenshot that clearly exhibits you what “tableHeader.js” seems like in a browser:
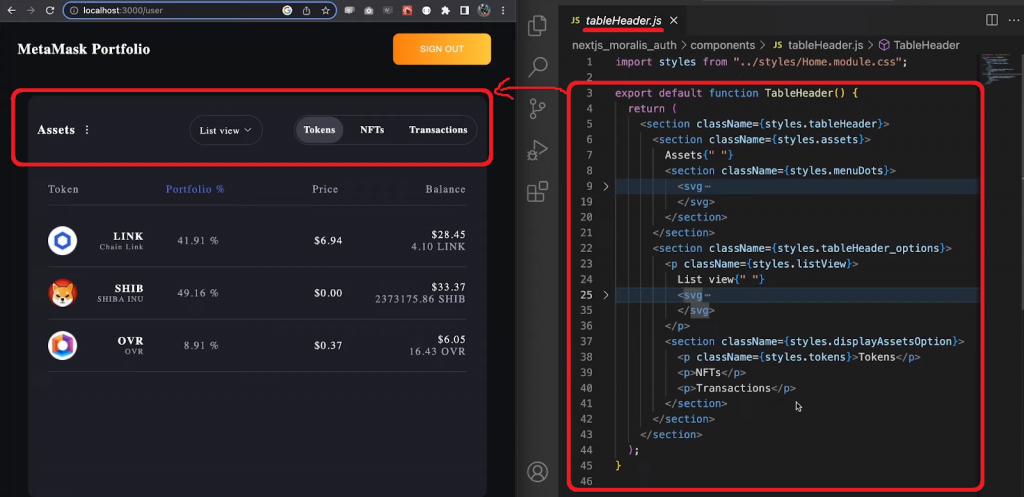
As you may see, the “tableHeader.js” script primarily offers with formatting and styling, which isn’t the purpose of this tutorial. The identical is true for the “tableContent.js” element, which ensures that the desk columns match our targets. It additionally calls the “GetWalletTokens” element:
The “getWalletTokens.js” script will get the on-chain knowledge fetched by the backend. So, are you questioning get blockchain knowledge from the backend to frontend? The “getWalletTokens.js” script makes use of useAccount from the wagmi library. The latter extracts the linked tackle and prompts the backend server with that tackle by way of Axios:
useEffect(() => {
let response;
async operate getData() {
response = await axios
.get(`http://localhost:5001/gettokens`, {
params: { tackle },
})
.then((response) => {
console.log(response.knowledge);
setTokens(response.knowledge);
});
}
getData();
}, []);
Trying on the above code snippet, you may see that the response variable shops all of the on-chain knowledge that the backend fetched. The script additionally saves response.knowledge within the setTokens state variable. Then, the return operate contained in the “getWalletTokens.js” script renders the ultimate element: “card.js“:
return (
<part>
{tokens.map((token) => {
return (
token.usdPrice && (
<Card
token={token}
whole={tokens[3]}
key={token.walletBalance?.image}
/>
)
);
})}
</part>
);
Whereas rendering “card.js“, “getWalletTokens.js” passes alongside some props. The “card.js” element then makes use of these props to render the small print that lastly populate the “Property” desk:
Remaining Construct of Our Polygon Portfolio Tracker
When you efficiently run the above-presented backend and frontend, you’ll be capable to check your tracker on “localhost:3000“:
Because the above screenshot signifies, you have to first join your MetaMask pockets by clicking on the “Join MetaMask” button.
Notice: By default, MetaMask doesn’t embrace the Polygon community. Thus, be certain so as to add that community to your MetaMask and change to it:
When you join your pockets, your occasion of our portfolio tracker dapp will show your tokens within the following method:
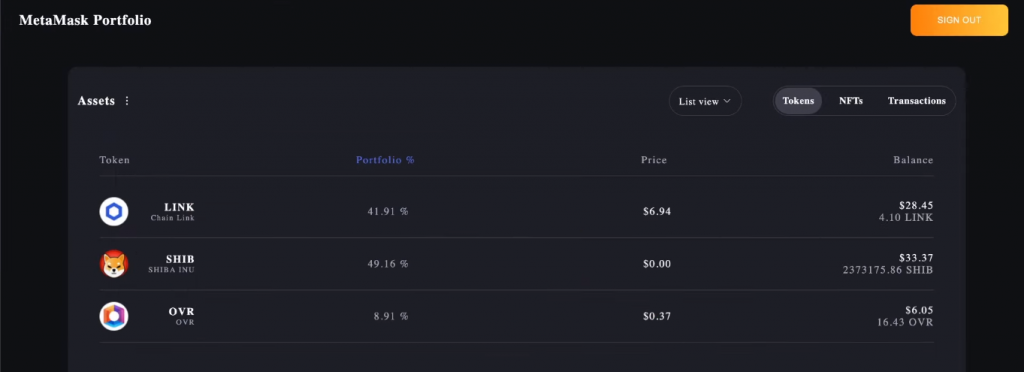
Notice: The “Tokens” part of our desk is the one one at present energetic. Nonetheless, we urge you to make use of the Moralis assets so as to add performance to the “NFTs” and “Transactions” sections as effectively.
Past Polygon Portfolio Tracker Improvement
Should you took on the above tutorial, you most likely keep in mind that our instance dapp solely makes use of two Moralis Web3 Knowledge API endpoints. Nonetheless, many different highly effective API endpoints are at your disposal while you go for Moralis. Whereas Moralis focuses on catering Web3 wallets and portfolio trackers, it may be used for all kinds of dapps.
Your entire Moralis fleet encompasses the Web3 Knowledge API, Moralis Streams API, and Authentication API:
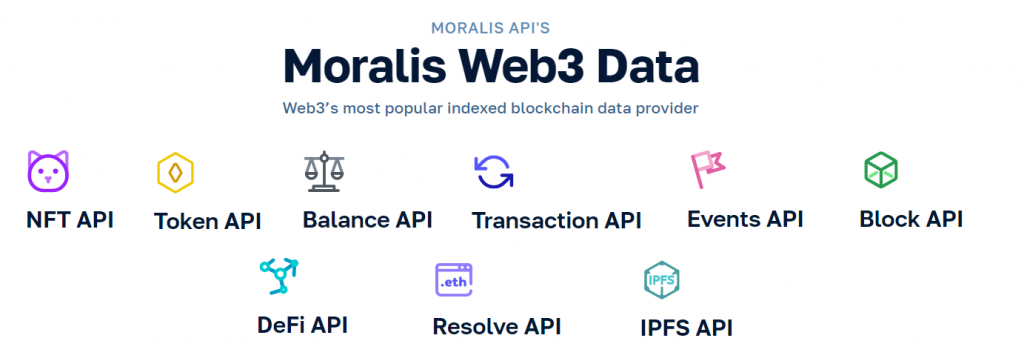
The Web3 Knowledge API comprises the next APIs enabling you to fetch any on-chain knowledge the straightforward method: NFT APIToken APIBalances APITransaction APIEvents APIBlock APIDeFi APIResolve APIIPFS API
With the Moralis Streams API, you may hearken to real-time on-chain occasions for any good contract and pockets tackle. That method, you should use on-chain occasions as triggers to your dapps, bots, and so on. Plus, you should use the facility of Moralis Streams by way of the SDK or our user-friendly dashboard.
Due to the Moralis Web3 Authentication API, you may effortlessly unify Web3 wallets and Web2 accounts in your functions.
Notice: You may discover all of Moralis’ API endpoints and even take them for check rides in our Web3 documentation.
All these instruments assist all of the main blockchains, together with non-EVM-compatible chains, corresponding to Solana and Aptos. As such, you’re by no means caught to any explicit chain when constructing with Moralis. Plus, due to Moralis’ cross-platform interoperability, you should use your favourite legacy dev instruments to affix the Web3 revolution!
Apart from enterprise-grade Web3 APIs, Moralis additionally gives another helpful instruments. Two nice examples are the gwei to ether calculator and Moralis’ curated crypto faucet listing. The previous ensures you by no means get your gwei to ETH conversions mistaken. The latter supplies hyperlinks to vetted testnet crypto taps to simply get hold of “check” cryptocurrency.
Find out how to Construct a Polygon Portfolio Tracker – Abstract
In at present’s article, you had a chance to observe our lead and create your personal Polygon portfolio tracker dapp. By utilizing our scripts, you had been in a position to take action with minimal effort and in a matter of minutes. In any case, you solely needed to create your Moralis account, get hold of your Web3 API key and retailer it in a “.env” file, set up the required dependencies, substitute 0x1 with 0x89, and run your frontend and backend. You additionally had an opportunity to deepen your understanding by exploring crucial scripts behind our instance portfolio tracker dapp. Final however not least, you additionally realized what Moralis is all about and the way it could make your Web3 improvement journey an entire lot less complicated.
When you’ve got your personal dapp concepts, dive straight into the Moralis docs and BUIDL a brand new killer dapp. Nonetheless, if you have to increase your blockchain improvement data first or get some attention-grabbing concepts, be certain to go to the Moralis YouTube channel and the Moralis weblog. These locations cowl all kinds of matters and tutorials that may provide help to change into a Web3 developer free of charge. For example, you may discover the superior Alchemy NFT API different, the main Ethereum scaling options, learn to get began in DeFi blockchain improvement, and rather more. Furthermore, on the subject of tutorials, you may select quick and easy ones because the one herein, or you may deal with extra intensive challenges. A terrific instance of the latter could be to construct a Web3 Amazon clone.
Nonetheless, when you and your workforce want help scaling your dapps, attain out to our gross sales workforce. Merely choose the “For Enterprise” menu possibility, adopted by a click on on the “Contact Gross sales” button:
[ad_2]
Source link