[ad_1]
Superior sensible contract programming isn’t any stroll within the park. Nonetheless, in case you deal with standardized sensible contracts, you possibly can deploy them in minutes. How is that doable? By importing OpenZeppelin contracts in Remix, which is what we’ll reveal on this article. Now, to make use of Remix to import OpenZeppelin contracts, a single line of code does the trick most often. For instance, to create an ERC20 token, the next line of code introduces a verified sensible contract template from OpenZeppelin in Remix:
import “https://github.com/OpenZeppelin/openzeppelin-contracts/blob/grasp/contracts/token/ERC20/ERC20.sol”;
If you happen to want to discover ways to create a easy ERC-20 sensible contract that makes use of the above line of code, ensure that to make use of the video above or comply with our lead within the “OpenZeppelin ERC-20 Instance” part beneath. As well as, in case you want to begin constructing dapps, similar to Web3 wallets and portfolio trackers that may fetch on-chain information and take heed to current sensible contracts, create your free Moralis account and begin BUIDLing!

Overview
The core of right now’s article can be our tutorial on creating an ERC-20 token. As we accomplish that, we’ll make the most of MetaMask, Remix, and OpenZeppelin. MetaMask will affirm the on-chain transaction required to deploy our ERC-20 contract on the Goerli testnet. That is the place it pays to know easy methods to get Goerli ETH from a dependable take a look at ether faucet. With a adequate quantity of Goerli ETH, you’re going to get to cowl transaction fuel and, in flip, full the “create ERC-20 token” feat. Nonetheless, it’s essential to first use Remix to import OpenZeppelin and add the required strains of code to create a easy sensible contract. Primarily, this can be a beginner-friendly sensible contract programming tutorial.
Within the second a part of right now’s article, we’ll present extra context surrounding the subject of importing OpenZeppeling contracts in Remix. As such, we’ll discover Remix, OpenZeppelin, and sensible contracts and reply the “what’s OpenZeppelin?” and “what’s the Remix IDE?” questions.
Lastly, we’ll look past sensible contracts and the instruments required to write down and deploy them. In spite of everything, you possibly can create killer dapps based mostly on current sensible contracts. That is the place Moralis – the main Web3 API supplier – simplifies Web3 growth. We’ll even present you considered one of Moralis’ final Token API endpoints in motion – we’ll fetch our instance ERC-20 token’s metadata.
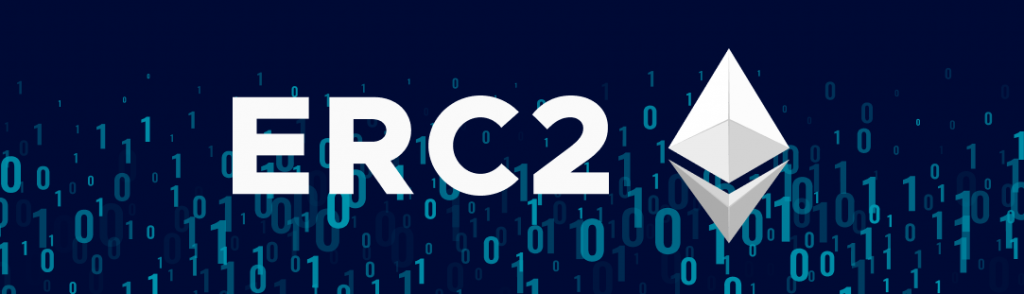
OpenZeppelin ERC-20 Instance – Learn how to Import OpenZeppelin Contracts in Remix
To finish this OpenZeppelin ERC-20 instance, ensure that to have the aforementioned instruments prepared. You must have a MetaMask net browser plugin put in and topped with some Goerli ETH. Now, in case you need assistance with that, use the “easy methods to get Goerli ETH” hyperlink within the overview part. With that stated, it’s time to indicate you easy methods to import OpenZeppelin contracts and deploy them with Remix.
Earlier than you can begin importing OpenZeppelin sensible contracts in Remix, it is advisable know easy methods to entry Remix and create a brand new venture. So, use your browser (the one with the MetaMask extension) and open Remix. You’ll be able to merely question Google for “Remix IDE”:
As soon as on the Remix venture web page, click on on the “IDE” possibility within the prime menu. Then, choose “Remix On-line IDE”:
As soon as on the Remix IDE house display, deal with the left sidebar. That is the place you possibly can choose the default “contracts” folder and use the “new file” icon to create a brand new sensible contract file:
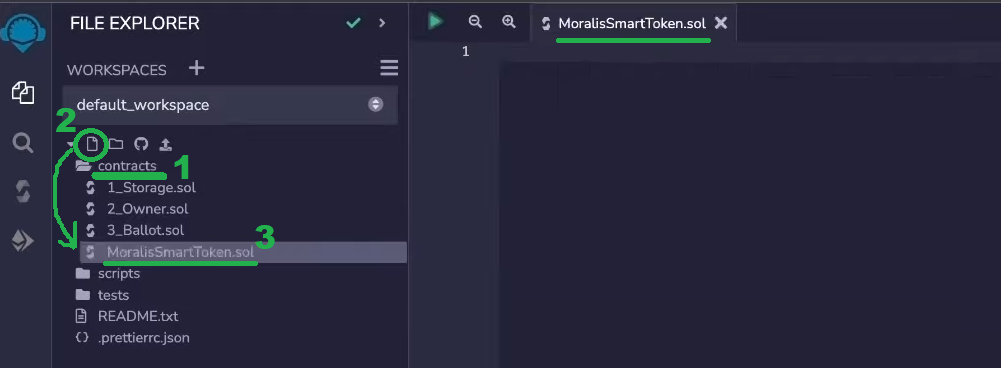
In the case of naming your sensible contract script, you should utilize your personal title or comply with our lead and go together with “MoralisSmartToken.sol”. Along with your new “.sol” file prepared, it’s time to populate it with the required strains of code. So, let’s present you the way easy our OpenZeppelin ERC-20 instance is.
OpenZeppelin in Remix – Utilizing a Verified ERC-20 Good Contract
The primary line in each Solidity sensible contract defines the model of Solidity. So, that is the code snippet that focuses on the “0.8.0” model:
//SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
The “^” image earlier than the model quantity indicators that every one newer Solidity variations may also work with our sensible contract. After the pragma line, we have now a spot to import OpenZeppelin in Remix. There are a number of methods to do that, however we’ll use the GitHub hyperlink, as offered earlier. Therefore, our instance contract’s subsequent line is the next:
import “https://github.com/OpenZeppelin/openzeppelin-contracts/blob/grasp/contracts/token/ERC20/ERC20.sol”;
By importing OpenZeppelin contracts in Remix, all capabilities and logic of imported contracts develop into out there in your script. So, when you import the above ERC-20 contract, you will have all it is advisable create your ERC-20 token.
Outline Contract, Use Constructor, and Name the “Mint” Perform
Beneath the import line, specify a brand new contract. Once more, be happy to make use of the identical title as we do (“MoralisSmartToken”) or select your personal title. That is the road of code that defines the brand new contract:
contract MoralisSmartToken is ERC20 {
Subsequent, we have to add a constructor, which is a particular form of perform that will get referred to as the primary time a wise contract is deployed to the community. In the case of an ERC-20 constructor, it wants to soak up two parameters: the token’s title and image. Nonetheless, as a substitute of hardcoding the precise title and image, you should utilize variables. That method, a person deploying the contract can select the title and the image. This can develop into clearer as soon as we present you easy methods to deploy our instance contract. Listed here are the strains of code it is advisable use to type a correct constructor:
constructor(string reminiscence _name, string reminiscence _symbol) ERC20(_name, _symbol) {
Inside any ERC-20 contract, there’s additionally the “mint” perform. The latter takes within the deal with to which the minted tokens ought to be despatched and the quantity of an ERC-20 token to be minted. Since importing OpenZeppelin contracts in Remix additionally imports their capabilities, you possibly can merely name the “mint” perform inside your constructor:
_mint(msg.sender, 1000 * 10 **18);
Within the line of code above, “msg.sender” is a world variable that refers back to the deal with that deploys the sensible contract. So far as the numbers contained in the “mint” perform go, they take care of the truth that Solidity doesn’t learn decimals (18 decimal locations) and can mint 1,000 tokens. This line can be the final line of our sensible contract.
Our OpenZeppelin ERC-20 Instance Script
Since you determined to make use of Remix to import an OpenZeppelin ERC-20 contract, the six strains of code lined above do the trick. Right here’s the entire “MoralisSmartToken.sol” script:
//SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import “https://github.com/OpenZeppelin/openzeppelin-contracts/blob/grasp/contracts/token/ERC20/ERC20.sol”;
contract MoralisSmartToken is ERC20 {
constructor(string reminiscence _name, string reminiscence _symbol) ERC20(_name, _symbol) {
_mint(msg.sender, 1000 * 10 **18);
}
}
Time to Deploy the Imported OpenZeppelin Contract in Remix
At this stage, you need to have the above strains of code in place. As such, you possibly can transfer past importing OpenZeppelin contracts in Remix. Nonetheless, earlier than you possibly can deploy your sensible contracts, it is advisable compile them, which is an easy two-click course of. You simply have to go to the “Solidity Compiler” tab and hit the “Compile [contract name]” button:
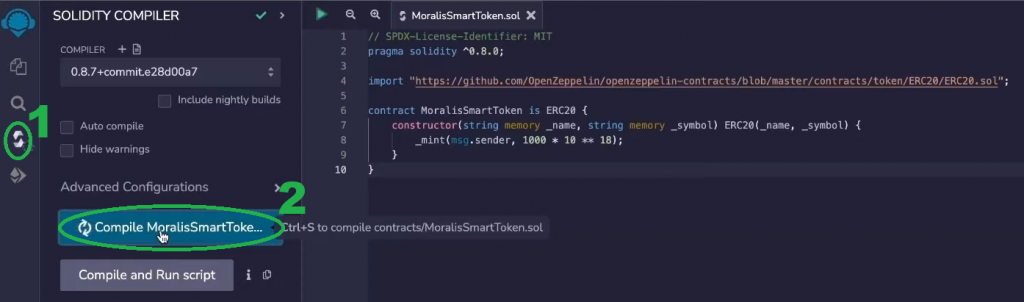
If there aren’t any errors in your code, the next content material seems within the facet menu beneath the “Compile” button:
After efficiently compiling your sensible contract, it’s time to deploy it. That is the place you’ll want your MetaMask pockets (related to the Goerli testnet) and topped with some Goerli ETH. As soon as on the “Deploy and Run Transaction” tab, choose “Injected Supplier-MetaMask”:
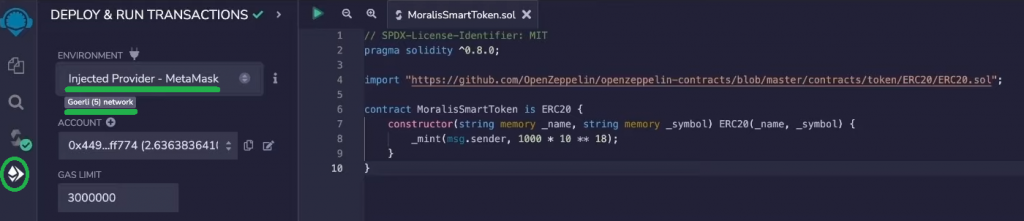
On the identical tab, there’s the “DEPLOY” part. The latter comes with a drop-down possibility the place you get to enter your token’s title and image. With these variables assigned, you possibly can click on on the “transact” button and ensure the associated transaction through the MetaMask module:
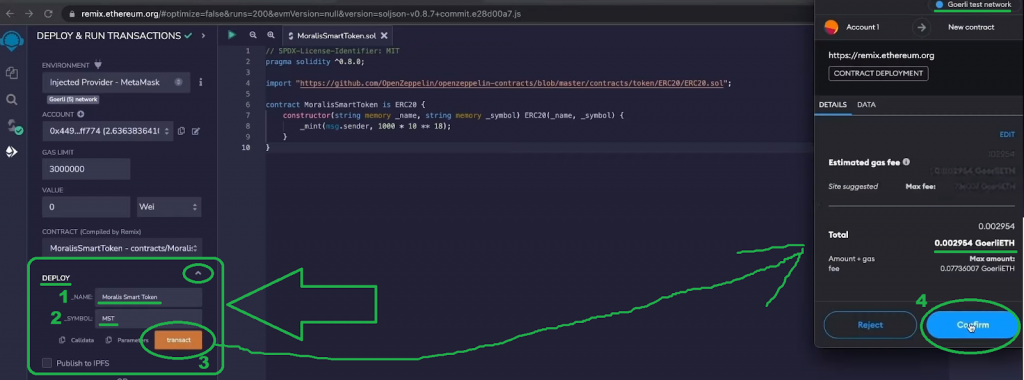
Add Your ERC-20 Token to MetaMask
As quickly because the above transaction is confirmed, you possibly can add your new ERC-20 token to your MetaMask. To do that, copy deployed contract’s deal with on the backside of the “Deploy” tab within the Remix IDE:
Then, open your MetaMask, choose the “Belongings” tab and click on on the “Import tokens” possibility:
On the “Import tokens” web page, paste the above-copied contract deal with within the designated entry discipline. This can robotically populate the “image” and “decimals” fields. Lastly, hit the “Add customized token” button:
That’s how simple it’s to create and deploy an ERC-20 token utilizing an OpenZeppelin contract in Remix! Now, in case you’d wish to dive into Remix, OpenZeppelin, and sensible contracts additional, learn on!
Exploring Remix, OpenZeppelin, and Good Contracts
To know easy methods to import OpenZeppelin contracts, you don’t should be an knowledgeable concerning the idea. Nonetheless, understanding the fundamentals normally makes issues clearer. As such, let’s briefly cowl Remix, OpenZeppeling, and sensible contracts.
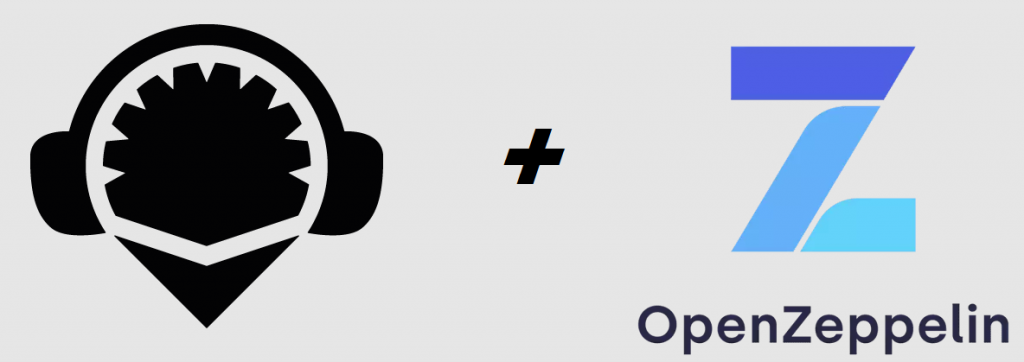
What are Remix and OpenZeppelin?
“Remix” is mostly used to check with the Remix IDE (built-in growth setting). The latter is an open-source growth setting that comes within the type of an internet and desktop software. The Remix IDE permits devs to cowl the complete strategy of sensible contract growth for Ethereum and different EVM-compatible chains. Moreover, “Remix” may additionally check with “the Remix venture” – a platform for plugin structure growth instruments. The main sub-projects of the Remix venture are the IDE, plugin engine, and Remix Libs. If you wish to discover the Remix IDE in additional element, use the “what’s the Remix IDE?” hyperlink within the overview of right now’s article.
OpenZeppelin is an open-source platform for constructing safe dapps, with a framework offering the required instruments to create and automate Web3 functions. OpenZeppelin additionally has sturdy audit companies, which high-profile prospects such because the Ethereum Basis and Coinbase depend on. Except for conducting safety audits on demand and implementing safety measures to make sure that your dapps are safe, OpenZeppelin has different instruments/companies. For example, OpenZeppelin Defender is an internet software that may, amongst many issues, safe and automate sensible contract operations. However what the vast majority of builders worth probably the most is OpenZeppelin’s intensive library of modular and reusable contracts, considered one of which we utilized in right now’s ERC-20 instance. In case you need to dive deeper into the “what’s OpenZeppelin?” subject, use the matching hyperlink within the overview.
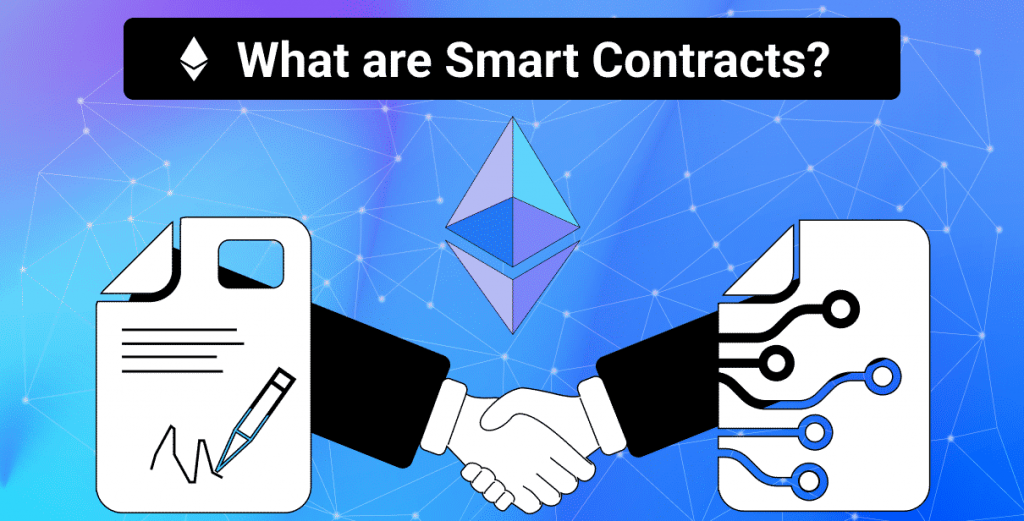
What are Good Contracts?
Good contracts are on-chain applications – items of software program saved on programmable blockchains similar to Ethereum. Good contracts are all about reinforcing predefined guidelines and automation. Based on the code behind every sensible contract, the latter executes predetermined actions when predefined circumstances are met. As such, it’s apparent that requirements have to be set to make sure these on-chain applications’ validity, efficacy, and effectivity. One such customary is ERC-20, which focuses on fungible tokens. Nonetheless, there are numerous different requirements, and by importing OpenZeppeling contracts in Remix, you possibly can adjust to all ERC requirements. If you happen to’d wish to discover two different token requirements, ensure that to learn our “ERC721 and ERC1155” article.
Past Remix and OpenZeppelin
Whether or not you resolve to make use of Remix to import OpenZeppelin and deploy your personal sensible contract or to deal with current sensible contracts, the secret’s to convey the ability of automation to the general public. That is the place dapps play probably the most very important position. Furthermore, now that you know the way to import OpenZeppelin contracts, it’s time you begin constructing dapps. Not way back, you’d should run your personal RPC node and construct your personal infrastructure to create an honest dapp. However issues have come a good distance, and legacy devs can now simply be part of the Web3 revolution, and Moralis is arguably the perfect Web3 supplier!
With a free account, you possibly can entry all of Moralis’ merchandise:
Since all of the highly effective instruments outlined above work on all main blockchains, Moralis offers you a broad attain and future-proofs your work. Moralis can be all about cross-platform interoperability, enabling you to make use of your favourite Web2 programming languages, frameworks, and platforms to create killer dapps. To take action, these are the steps to take:
Use Remix to import OpenZeppelin and deploy your sensible contracts or deal with current ones.Use Moralis to construct dapps, similar to Web3 wallets and portfolio trackers, enabling folks to work together with sensible contracts and Web3 in a user-friendly method.
Token API Endpoint Instance
In gentle of right now’s “OpenZeppelin in Remix” tutorial, let’s have a look at the “getTokenMetadata” endpoint for instance. Simply by accessing this endpoint’s reference web page in Moralis’ docs, we will get any token’s metadata by selecting a sequence and inserting a contract deal with:
Listed here are the outcomes for the above-created “MST” token:
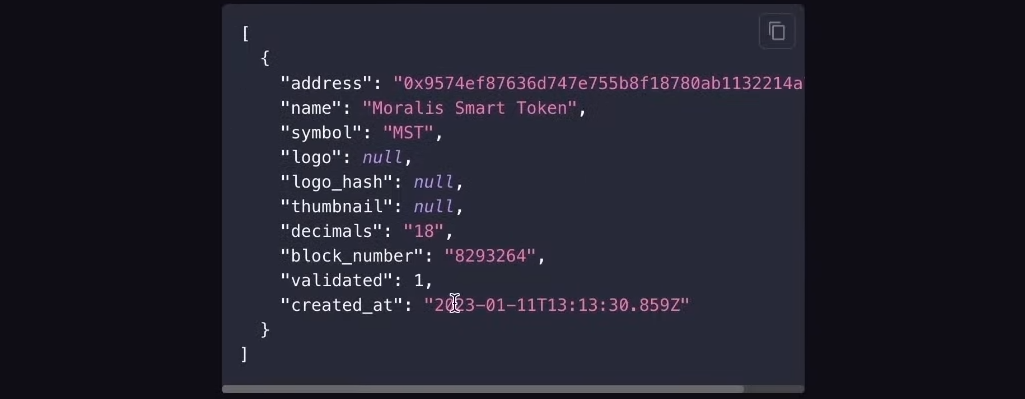
Now that you know the way to import OpenZeppelin contracts, you possibly can simply use the ability of Moralis to focus on your contracts. Importing OpenZeppelin contracts in Remix or utilizing every other various, begin BUIDLing right now with Moralis!
OpenZeppelin in Remix – Importing OpenZeppelin Contracts in Remix – Abstract
In right now’s article, we took you by the hand, exhibiting you easy methods to import OpenZeppelin contracts whereas specializing in an OpenZeppelin ERC-20 instance. You now know that importing OpenZeppelin contracts in Remix is so simple as pasting a correct GitHub deal with proper after the “import” line. Except for studying easy methods to use Remix to import OpenZeppelin, you additionally realized easy methods to compile and deploy sensible contracts utilizing the Remix IDE. Transferring past our “OpenZeppelin in Remix” tutorial, we additionally defined what OpenZeppelin, Remix, and sensible contracts are. Final however not least, you additionally realized the gist of Moralis. As such, you at the moment are prepared to begin constructing dapps round your or current sensible contracts.
Following right now’s ERC-20 instance, you might simply create an ERC-721 token or ERC-1155 NFTs. In each instances, you’d import OpenZeppelin in Remix however as a substitute deal with the ERC-721 or ERC-1155 contract templates. Additionally, throughout your growth endeavors, it’s possible you’ll want different helpful instruments, similar to a dependable gwei to ETH calculator or a vetted crypto faucet.
Moreover, it’s possible you’ll be considering exploring different blockchain growth subjects. If that’s the case, ensure that to take a look at the Moralis YouTube channel and the Moralis weblog. Amongst many different subjects, these are the locations to be taught extra in regards to the Solana Python API and the main Solana testnet faucet. In case you’d like a extra skilled strategy to your crypto schooling, take into account enrolling in Moralis Academy. There you can begin with Ethereum fundamentals or attend extra superior programs.
[ad_2]
Source link